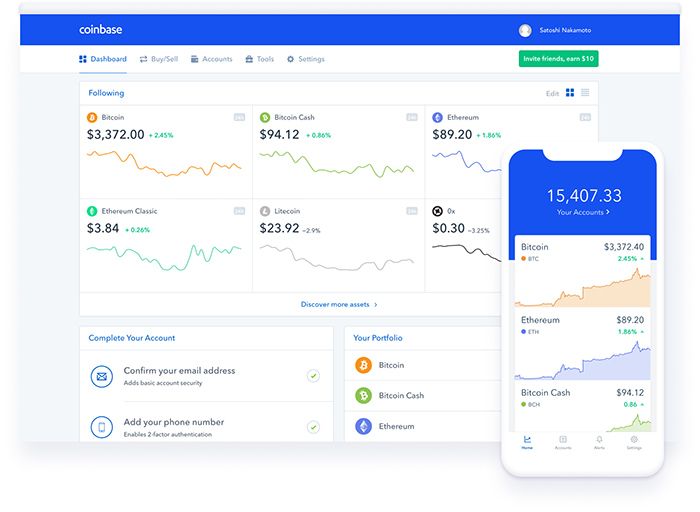
CLI Data Gem 🍻
It's that time... time for my first project at Flatiron Software Engineering Bootcamp. The task at hand was to leverage all we have learned about Ruby and object oriented programming in order to build an OO ruby application that uses the CLI. Simple enough, right? Oh and just for fun, we are required to scrape an external data source as part of it.
Before diving into my project, I figured I would toss out three things I learned in the process of completing this assignment.
- Having a plan will save you time. As someone who builds furniture from Ikea without the instructions (anyone else?)... this was a necessary lesson for me to learn. It wasn't that I couldn't think of the logical steps on the fly, it was that I didn't realize how necessary a frame of reference would be in the wee hours of the night or after a long day at work. My chicken scratch diagram was invaluable and I will be sure to start future projects with this in mind.
- Be mindful of the sites you are scraping. Only scrape what is necessary and be specific. No need to DDOS (hyperbole) the site that is so generously providing you data!
binding.pry
is your friend and you should hang out more! A lot more.
The Project
Requirements for the project are as follows:
- Provide a CLI
- Your CLI application must provide access to data from a web page.
- The data provided must go at least one level deep. A "level" is where a user can make a choice and then get detailed information about their choice.
- Use good OO design patterns.
Coinbase sounds like fun
Coinbase allows users to buy, sell and manage their cryptocurrency portfolio. I decided to create an application that would allow a user to see what price specific cryptocurrencies were trading for at the time the application executed. My "second level" would ask a user to select a coin using the coin's short-code (i.e BTC
) which should return the coin's market cap, description and landing page URL.
The application consisted of three main pieces. The Scraper Class
, the Coin Class
, and the CLI Class
. After I had my file structure setup, I proceeded to building the scraper. I used pry to return results to the terminal to verify I was consuming the right data. I have two methods in this Class. My first method scrapes the main Coinbase page for the names of the coins, the price, the market cap and each coin's individual Coinbase page URL. The descriptions of the coins can be found on the individual pages for each coin (why the URL is important to store). Rather than grabbing all of this in one scrape, I am waiting until the user selects a coin to specifically go scrape for that Coin's description. My initial coin list is created by passing an array from the first scrape into the Coin class.
My coin class is responsible for creating the Coin objects and assigning them attributes. The attributes I assigned were the things above I needed to scrape. I had to write a few of methods to interact with the Coin objects. I needed to find a specific coin object from the list of Coin.all
using the shortcode
. I also needed to update a Coin with the description
once the user had selected that coin.
Finally, I needed to design how the user would interact with the CLI in order to get the data they needed. This part was fun and I still have some wish list items I hope to add. The flow is fairly simple. I show the user a list of coins, allow them to pick a coin via short-code to see more detail and at that time allow them to start over or exit. After I had this working, I went back through my input = gets
and made sure I was considering edge cases where the user enters things I wasn't asking for and handled errors appropriately. I also made sure there was a clear way to exit the program within each prompt.
The next step for me is to make this a Ruby Gem and publish it at rubygems.org. This is just a "nice to have" step for me and a process I want to be familiar with. Will update this article with the RubyGems.org link once I have it! But in the meantime...
👉🏻 Check out the project on GitHub
Trade some crypto! Visit Coinbase.com
Thanks for reading! Cheers!